Java Fundamentals
If you learn something new every day, you can teach something new every day.
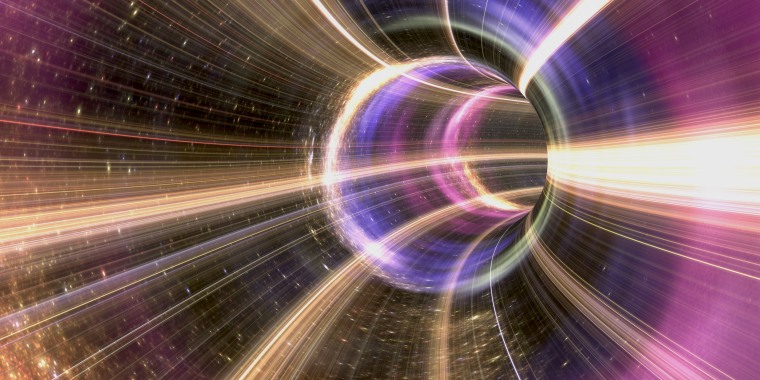
Subject: Java Fundamentals
Topics:
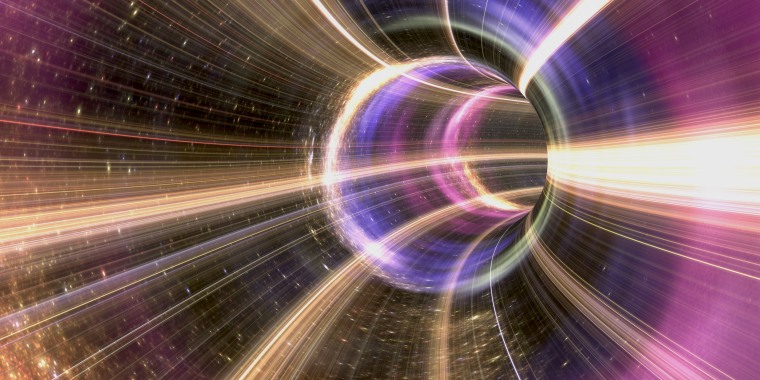
Subject: Java Fundamentals
Topics:
- Verifying Java JDK installation is correct or not from command line.
- Installation of Eclipse IDE
- Creating a Simple Java Application. (Hello World Program)
/**
* This class implements the HelloWorld program
* @author srinivas.kadiyala
* @version 1.0
*
*/
public class HelloWorld {
/*
* Using comments in HelloWorld
*/
public static void main(String[] args)
{
//Hello World Program
System.out.println("Hello World Again");
//Hello World Output - Spaces within the braces
System.out.println( "Hello World before Space in braces");
//Hello World Output - Spaces outside the spaces
System.out.println("Hello world before space outside braces") ;
//Hello World Output - New Line and Spaces within the braces
System.out.println(
"Hello World"
);
//Commenting out the program.
//System.out.println("Hello World Last Time");
}
}
- Run the program from Eclipse
- Run the Program from Command Line.
Step 1: Compilation of Java Code. - Successful.
Compiled without any errors.
Step 2: Running the Program.
Error: Could not find or load main class HelloWorld.
Tried different ways to make it correct. But after few minutes, with help of stackoverflow. Performed Step 3.
Step 3: Running the Program.
D:> java -cp . HelloWorld
-cp . means referring current classpath.
Program ran successfully and displayed the Output.